Merge pull request #18 from Nooo37/playerctl
New Playerctl Signal Module
This commit is contained in:
commit
8e76f6bcc6
53
README.md
53
README.md
|
@ -205,6 +205,54 @@ bling.module.tabbed.iter() -- iterates through the currently focused
|
|||
bling.module.tabbed.pick_with_dmenu() -- picks a client with a dmenu application (defaults to rofi, other options can be set with a string parameter like "dmenu")
|
||||
```
|
||||
|
||||
##### 🎵 Playerctl
|
||||
|
||||
This is a signal module in which you can connect to certain bling signals to grab playerctl info. Currently, this is what it supports:
|
||||
|
||||
- Song title and artist
|
||||
- Album art (the path this module downloaded the art to)
|
||||
- If playing or not
|
||||
- Position
|
||||
- Song length
|
||||
|
||||
This module relies on `playerctl` and `curl`. If you have this module disabled, you won't need those programs.
|
||||
|
||||
To enable: `bling.signal.playerctl.enable()`
|
||||
|
||||
###### Signals
|
||||
|
||||
```lua
|
||||
-- bling::playerctl::status -- first line is the signal
|
||||
-- playing (boolean) -- indented lines are function parameters
|
||||
-- bling::playerctl::album
|
||||
-- album_art (string)
|
||||
-- bling::playerctl::title_artist
|
||||
-- title (string)
|
||||
-- artist (string)
|
||||
-- bling::playerctl::position
|
||||
-- interval_sec (number)
|
||||
-- length_sec (number)
|
||||
```
|
||||
|
||||
###### Example Implementation
|
||||
|
||||
Lets say we have an imagebox. If I wanted to set the imagebox to show the album art, all I have to do is this:
|
||||
```lua
|
||||
local art = wibox.widget {
|
||||
image = "default_image.png",
|
||||
resize = true,
|
||||
forced_height = dpi(80),
|
||||
forced_width = dpi(80),
|
||||
widget = wibox.widget.imagebox
|
||||
}
|
||||
|
||||
awesome.connect_signal("bling::playerctl::album", function(path)
|
||||
art:set_image(gears.surface.load_uncached(path))
|
||||
end)
|
||||
```
|
||||
Thats all! You don't even have to worry about updating the imagebox, the signals will handle that for you.
|
||||
|
||||
|
||||
|
||||
### 🌈 Theme variables
|
||||
You will find a list of all theme variables that are used in bling and comments on what they do in the `theme-var-template.lua` file - ready for you to copy them into your `theme.lua`. Theme variables are not only used to change the appearance of some features but also to adjust the functionality of some modules. So it is worth it to take a look at them.
|
||||
|
@ -241,6 +289,11 @@ gif by [javacafe](https://github.com/JavaCafe01)
|
|||
|
||||
gif by me :)
|
||||
|
||||
### Playerctl Signals Implementation
|
||||
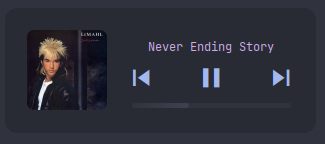
|
||||
|
||||
screenshot by [javacafe](https://github.com/JavaCafe01)
|
||||
|
||||
## TODO
|
||||
- [ ] Add external sources management for the wallpaper module (URLs, RSS feeds, NASA picture of the day, ...)
|
||||
- [ ] Scratchpad module
|
||||
|
|
7
init.lua
7
init.lua
|
@ -1,12 +1,9 @@
|
|||
--[[
|
||||
Bling
|
||||
Layouts, widgets and utilities for Awesome WM
|
||||
--]]
|
||||
|
||||
|
||||
return {
|
||||
--]] return {
|
||||
layout = require(... .. ".layout"),
|
||||
module = require(... .. ".module"),
|
||||
helpers = require(... .. ".helpers"),
|
||||
signal = require(... .. ".signal")
|
||||
}
|
||||
|
||||
|
|
|
@ -0,0 +1 @@
|
|||
return {playerctl = require(... .. ".playerctl")}
|
|
@ -0,0 +1,115 @@
|
|||
-- Provides:
|
||||
-- bling::playerctl::status
|
||||
-- playing (boolean)
|
||||
-- bling::playerctl::album
|
||||
-- album_art (string)
|
||||
-- bling::playerctl::title_artist
|
||||
-- title (string)
|
||||
-- artist (string)
|
||||
-- bling::playerctl::position
|
||||
-- interval_sec (number)
|
||||
-- length_sec (number)
|
||||
--
|
||||
local awful = require("awful")
|
||||
local beautiful = require("beautiful")
|
||||
|
||||
local interval = beautiful.playerctl_position_update_interval or 1
|
||||
|
||||
local function emit_player_status()
|
||||
local status_cmd = "playerctl status -F"
|
||||
|
||||
-- Follow status
|
||||
awful.spawn.easy_async_with_shell(
|
||||
"ps x | grep \"playerctl status\" | grep -v grep | awk '{print $1}' | xargs kill",
|
||||
function()
|
||||
awful.spawn.with_line_callback(status_cmd, {
|
||||
stdout = function(line)
|
||||
local playing = false
|
||||
if line:find("Playing") then
|
||||
playing = true
|
||||
else
|
||||
playing = false
|
||||
end
|
||||
awesome.emit_signal("bling::playerctl::status", playing)
|
||||
end
|
||||
})
|
||||
end)
|
||||
end
|
||||
|
||||
local function emit_player_info()
|
||||
local art_script = [[
|
||||
sh -c '
|
||||
|
||||
tmp_dir="$XDG_CACHE_HOME/awesome/"
|
||||
|
||||
if [ -z ${XDG_CACHE_HOME} ]; then
|
||||
tmp_dir="$HOME/.cache/awesome/"
|
||||
fi
|
||||
|
||||
tmp_cover_path=${tmp_dir}"cover.png"
|
||||
|
||||
if [ ! -d $tmp_dir ]; then
|
||||
mkdir -p $tmp_dir
|
||||
fi
|
||||
|
||||
link="$(playerctl metadata mpris:artUrl)"
|
||||
|
||||
curl -s "$link" --output $tmp_cover_path
|
||||
|
||||
echo "$tmp_cover_path"
|
||||
']]
|
||||
|
||||
-- Command that lists artist and title in a format to find and follow
|
||||
local song_follow_cmd =
|
||||
"playerctl metadata --format 'artist_{{artist}}title_{{title}}' -F"
|
||||
|
||||
-- Progress Cmds
|
||||
local prog_cmd = "playerctl position"
|
||||
local length_cmd = "playerctl metadata mpris:length"
|
||||
|
||||
awful.widget.watch(prog_cmd, interval, function(_, interval)
|
||||
awful.spawn.easy_async_with_shell(length_cmd, function(length)
|
||||
local length_sec = tonumber(length) -- in microseconds
|
||||
local interval_sec = tonumber(interval) -- in seconds
|
||||
if length_sec and interval_sec then
|
||||
if interval_sec >= 0 and length_sec > 0 then
|
||||
awesome.emit_signal("bling::playerctl::position",
|
||||
interval_sec, length_sec / 1000000)
|
||||
end
|
||||
end
|
||||
end)
|
||||
end)
|
||||
|
||||
-- Follow title
|
||||
awful.spawn.easy_async_with_shell(
|
||||
"ps x | grep \"playerctl metadata\" | grep -v grep | awk '{print $1}' | xargs kill",
|
||||
function()
|
||||
awful.spawn.with_line_callback(song_follow_cmd, {
|
||||
stdout = function(line)
|
||||
-- Get Album Art
|
||||
awful.spawn.easy_async_with_shell(art_script, function(out)
|
||||
local album_path = out:gsub('%\n', '')
|
||||
awesome.emit_signal("bling::playerctl::album",
|
||||
album_path)
|
||||
end)
|
||||
|
||||
-- Get Title and Artist
|
||||
local artist = line:match('artist_(.*)title_')
|
||||
local title = line:match('title_(.*)')
|
||||
awesome.emit_signal("bling::playerctl::title_artist", title,
|
||||
artist)
|
||||
end
|
||||
})
|
||||
end)
|
||||
end
|
||||
|
||||
-- Emit info
|
||||
-- emit_player_status()
|
||||
-- emit_player_info()
|
||||
|
||||
local enable = function()
|
||||
emit_player_status()
|
||||
emit_player_info()
|
||||
end
|
||||
|
||||
return {enable = enable}
|
Loading…
Reference in New Issue